Lessons learned from projects
What was learned over time for the projects.Large JS Chunks in Walcron website
Issues we faced- Page speed reported initial load JS for Walcron was large.
- Page load was slow when throttled with slow 3G.
Analysis did- Check on browser the file size loaded. Found one of the big chunk goes up-to 837kb.
- Ran `npm run analyze`, which was configured in next.config.js. Shows the big chunk was due to typescript ~902kb to load.
- The whole project was written in typescript, but strangely it should was imported and wasn' compiled! Then did a search and found a file importing the WHOLE typescript !
- Check alternative to find how 'eval' can be replaced. Unlike lodash, transpile is included only in typescript. Apparently eval can be replaced, it's actually Function.Global_Object
const evaluatedResult = Function(“"use strict";return ${mathEval}”)() return evaluatedResult
- Replaced code with Function and file size was fixed!
Testing with Object.definePropertyTest suite that have Object.definedProperty override on certain behaviours cannot be redefined. If test case runs after it's used hence it cannot be erased.
describe( "test", () => {
it("should one", ()=>{console.log(navigator.share)}) // = undefined
it("should two", ()=> {Object.defineProperty(window.navigator, "share"...})
it("should three", ()=>; {console.log(navigator.share)}) // = function ();
}
Jest Library Mock import must always be the firstJest mocked import for library order is important and must always be the first to override the import of the original 3rd party library.
import "../__mocked__/pusherMock"; //mock library must be the first.
import X from "someComponentThatUsesPusher"
--OR--
jest.mock('pusher', () => class Pusher{});
import Pusher from 'pusher'; //then only it takes effect.
Jest library with 3rd party library import under NEXT.JS is unsolvableError of `Cannot use import statement outside a module`, cannot be solved at the moment when 3rd party library are executed via mock. Only solution is to mock the whole library with jest.mock('whole library').
GIT commandshttps://www.internalpointers.com/post/squash-commits-into-one-git
git rebase --interactive HEAD~3
React SuspenseA new way to handle asynchronous response. But take notes on this:
- For it to work with NextJS, it needs to load via client side. Hence a need to use dynamic/lazy import.
- due to dynamic import, one will face a problem if directly routed of:A component suspended while responding to synchronous input. This will cause the UI to be replaced with a loading indicator. To fix, updates that suspend should be wrapped with startTransition.. To overcome it (sample in pageComponents/*/SnakeGame.tsx) it, simply wrap it in another Suspense tag
<Suspense><LazyLoadedComponent/></Suspense>
Google Tag- Click on a container and select Preview, find a button "Select Version" and do debugging. Do not use latest/live.
- Trigger some custom events sent to google tag. All google custom events sent with 'gtag(event)' requires manual configuration. To do so:
- Make sure codes sent a gtag event, e.g.
gtag("event", "CLS", {...metrics_id: 1})
//OR
gaLibrary.event("CLS", {...metrics_id: 1})
- Do a preview on the current page and to debug the event sent.
- Take note if "Tag Fired"; if it doesn't, meant something needs to be setup in google tag Trigger. Image below has INP configured to fire to GTM's GA.
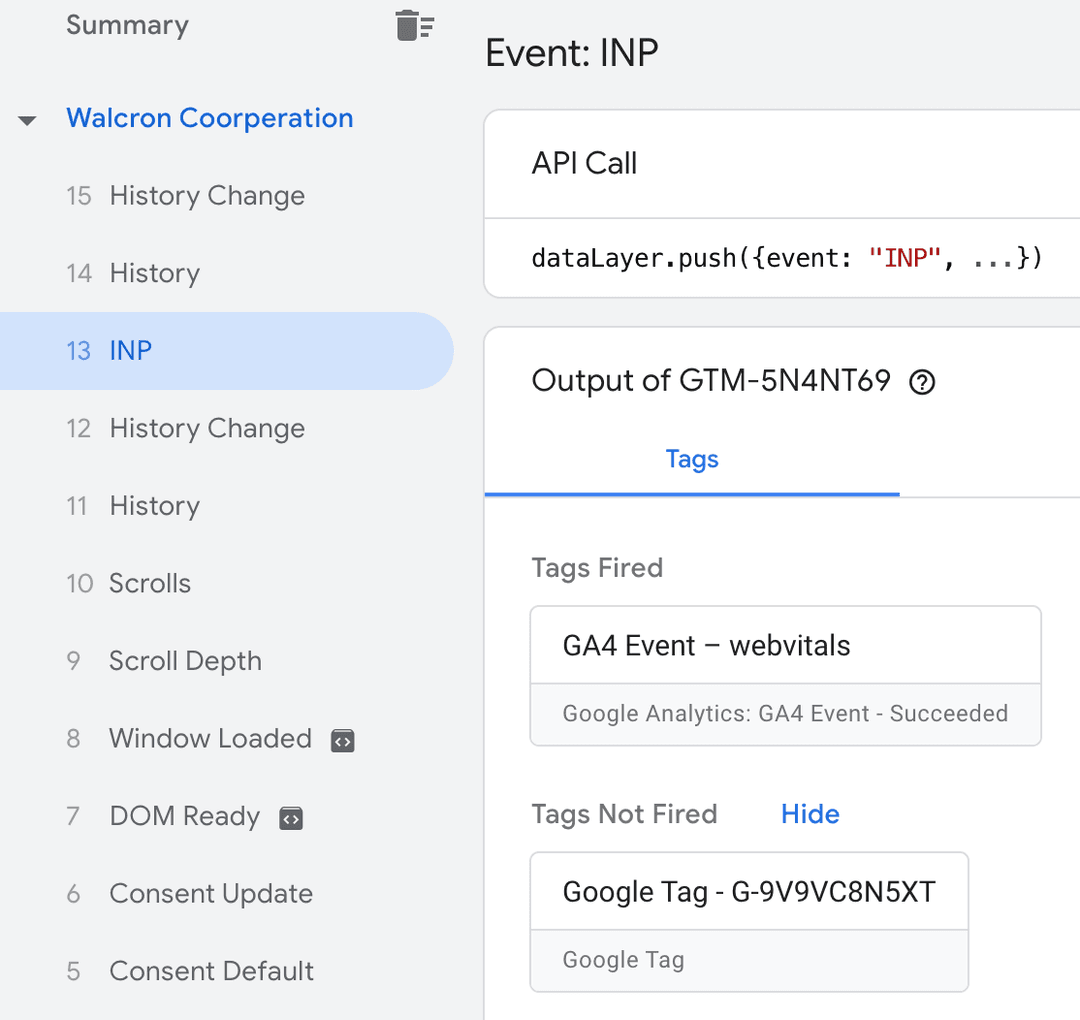
- Take note of the event model and this needs to be setup as well. In this example for INP event name model is:
eventModel { //take note this is using custom library, in pure gtag it is flat unless defined.
...
metric_delta: 72
sent_to: 'GTM...'
}
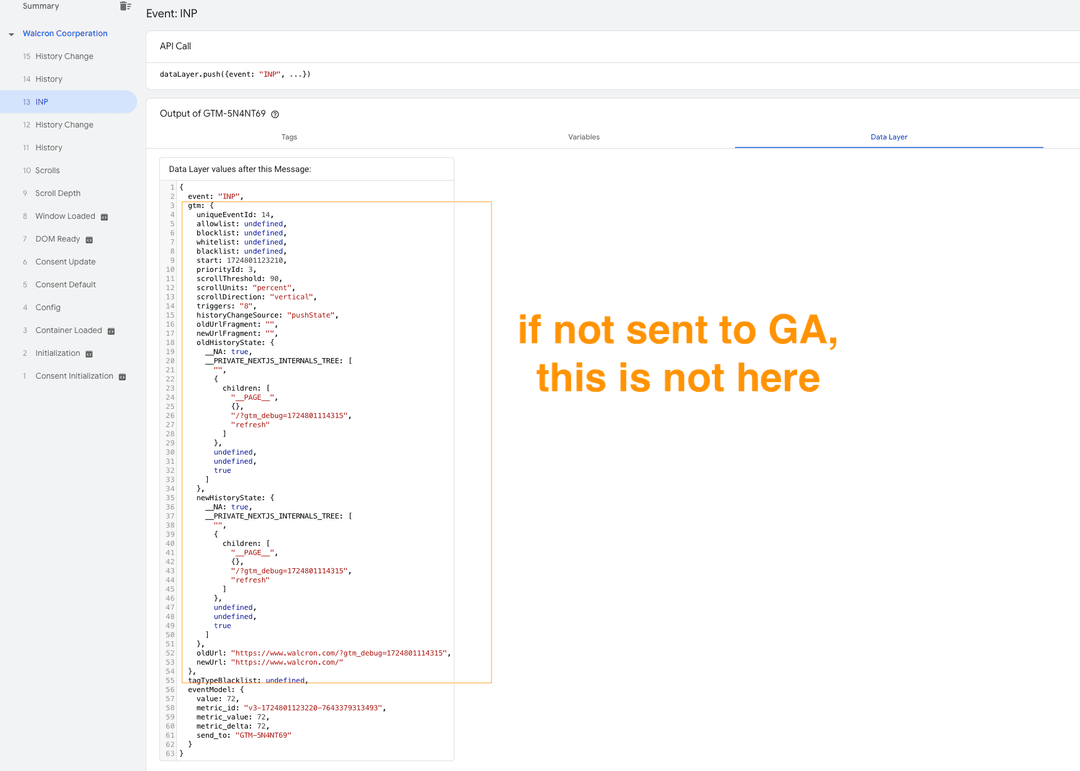
- Setup Google Tag
- Setup data variables matching the event model with Variables by selecting Select New in User Defined
- Create Data Layer Variable, and write the name similar to the model. As in this case "eventModel.metric.delta". Set as Version 2.
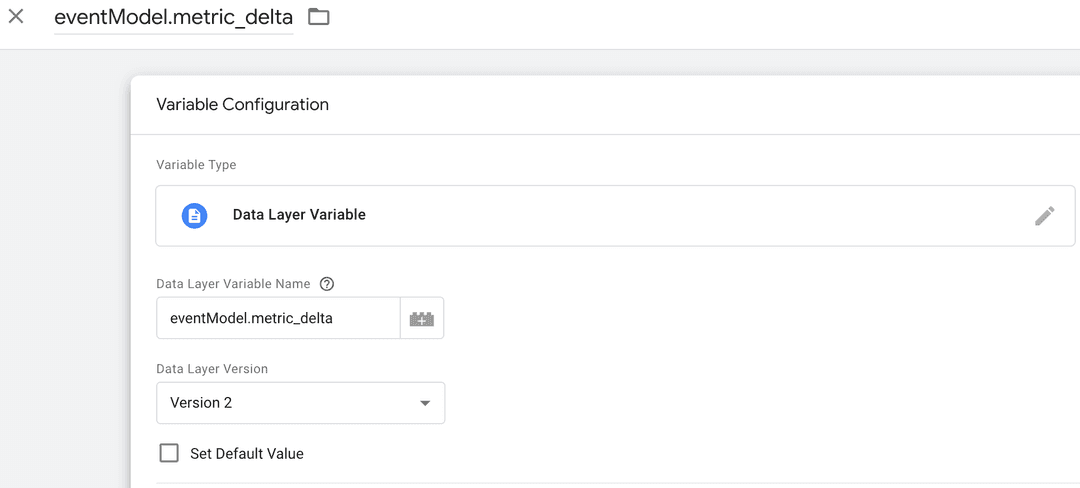
- Create a Trigger, name the Event name with a regex (without /) and make sure it triggers All Custom Events. Sample means matching all INP or CLS or LCP event name.
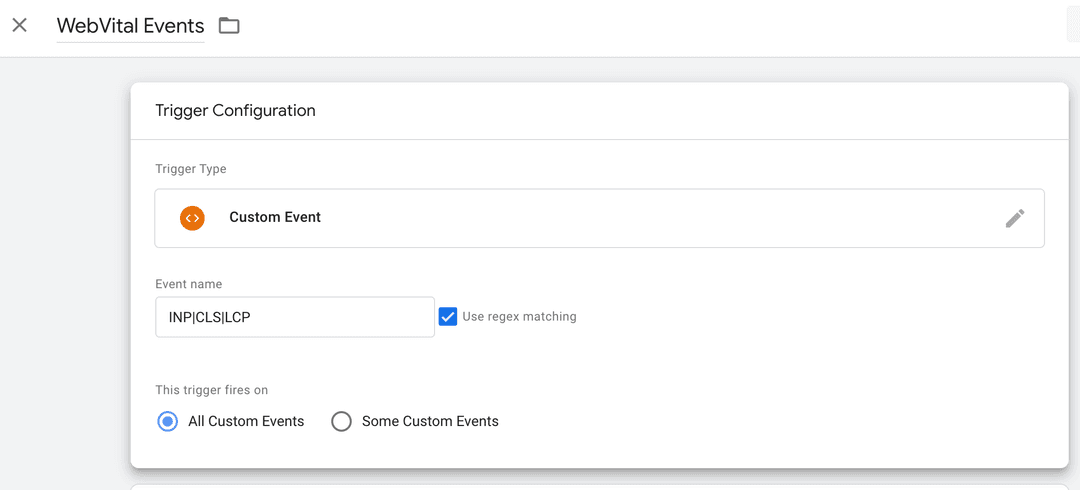
- Create a Tag. For the first step Tag the configuration as"Google Analytics: GA4 Event". Then:
- Get you measurement ID from google analytics -> Admin -> Data Collection and Modification -> Data Stream
- Use {{ Event }} as variable name instead of hardcoding. If hardcoded, all you custom event name will be the new name.
- Expand Event Parameters and map a new name to the data layer variable created. (Old version have a prefix of DLV)
- Add in consent with "No Additional Consent Required"
- Tie a trigger created with this Event
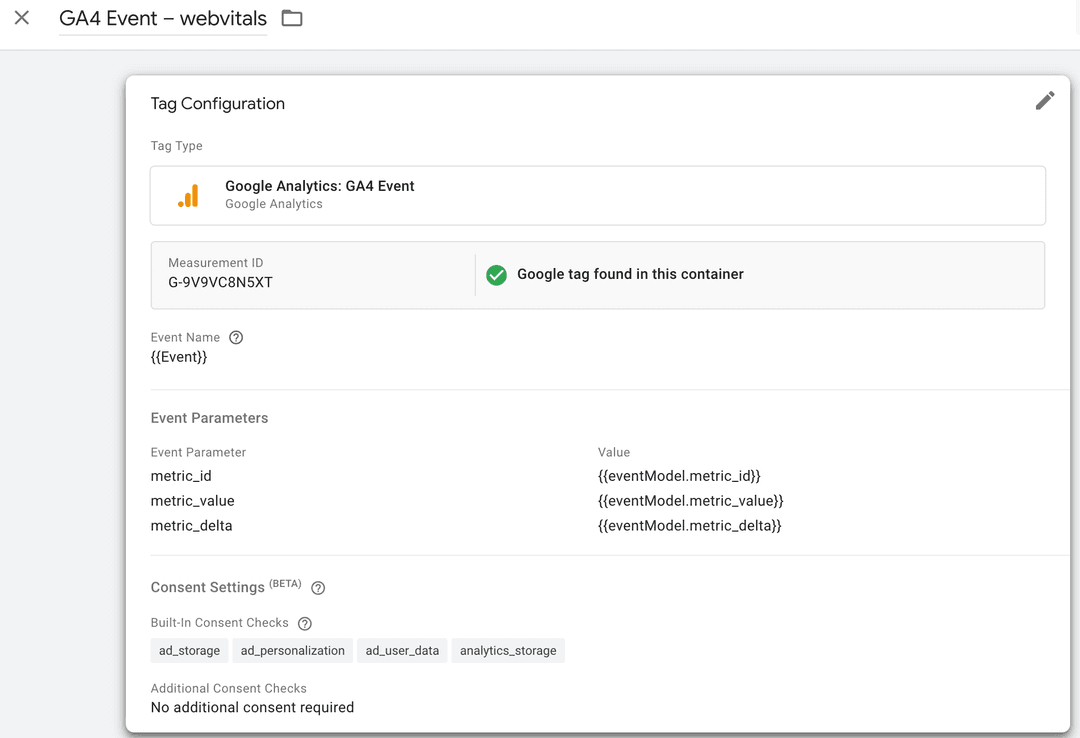
- Publish it then click Preview. During preview make sure debug is on and select "Version" of the newly published version.
Flex Justify CenterJustify center doesn't support overflow. Meaning if overflow is auto, the content gets snipped off.
To solve this, use margin auto with pseudo ::before and ::after. Refer to minimenu.
CI/CD Caveat
Github
- If one is creating actions, remember to add "shell: bash"for jobs running scripts.
- Remember to use "secrets: inherit" on parent job to enable secret passing to downstream workflow.
Domain Transfer from GoDaddy to CloudflareHad been a loyal customer of GoDaddy for 10 years, but price charges from them was insanely high - USD65 per year?!
Decided to move to Cloudflare which was only USD10/per year for the domain.
- Had change GoDaddy NS to Cloudflare and setup the domain here for quite some years.
- Login to Cloudflare and double check if walcron.com is listed in Domain Registration.
- Login to GoDaddy and make sure domainproxy email is forwarded.
- Initiate transfer from GoDaddy, then an authorization Code was emailed.
- Check the domain is unlocked in GoDaddy and browse WHOIS for walcron.com domain and make sure it is "Domain Status: ok http://icann.org/epp#ok"
- Goto Domain Transfer in Cloudflare, and fingers crossed it allows transfer. Might take up to a day.
- Trigger the transfer in Cloudflare and enter the Authorization Code.
- Fill-in and the form to Initiate a transfer. Once done, I received an email from GoDaddy.
- Follow the email of GoDaddy to approve the transfer
- WALA! All done. Best checked once more in Cloudflare and WHOIS to make sure domain is locked and secured.